Custom branding
This feature enables you to customize the look and feel of the Shake SDK. You can reflect your brand's identity by modifying colors, fonts, and other attributes of screen elements.
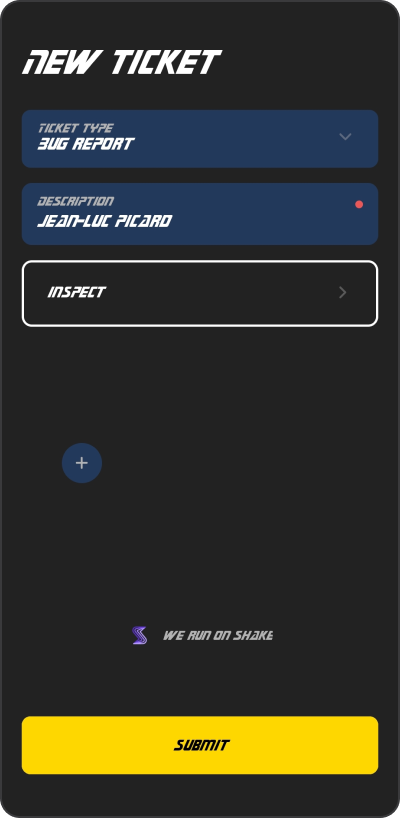
Star Trek style
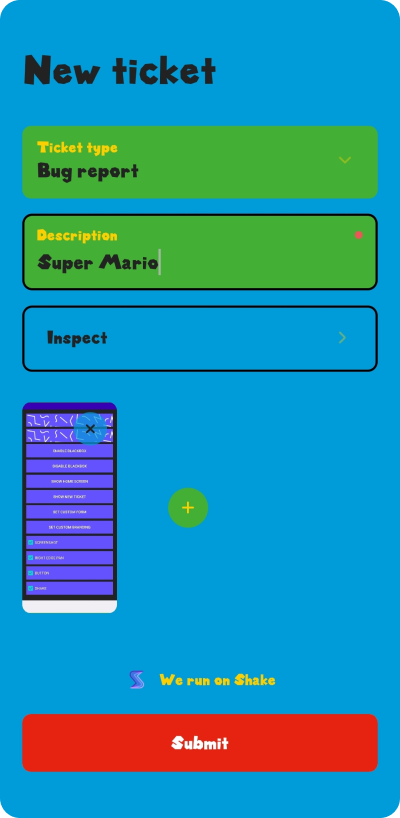
Super Mario vibes
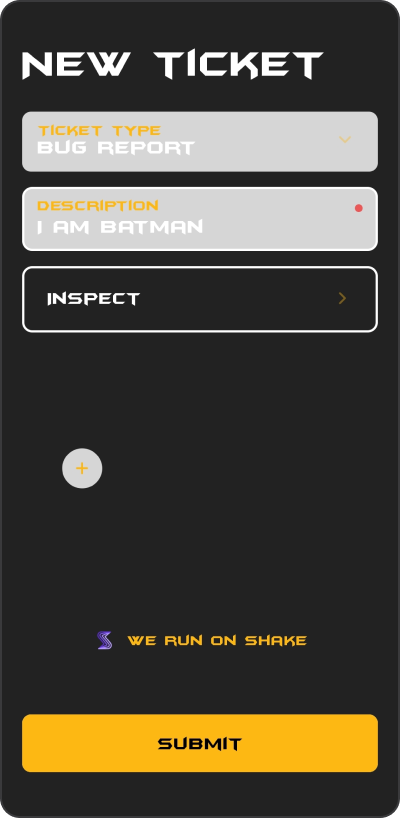
Batman UI
Setting up a custom theme
Use ShakeTheme instance to customize appearance of the Shake screens. Here is an example how you can create and set a new Shake theme:
ShakeTheme shakeTheme = ShakeTheme();shakeTheme.fontFamilyBold = 'assets/fonts/CoolFont-Bold.ttf';shakeTheme.fontFamilyMedium = 'assets/fonts/CoolFont-Regular.ttf';shakeTheme.backgroundColor = '#ffffff';shakeTheme.secondaryBackgroundColor = '#ffffff';shakeTheme.textColor = '#0e0e0e';shakeTheme.secondaryTextColor = '#3f3f3f';shakeTheme.accentColor = '#ff0000';shakeTheme.accentTextColor = '#ffffff';shakeTheme.outlineColor = '#464646';shakeTheme.borderRadius = 0.0;shakeTheme.elevation = 10.0; // Only AndroidshakeTheme.shadowOffset = Offset(0.1, 0.1); // Only iOSshakeTheme.shadowRadius = 3; // Only iOSshakeTheme.shadowOpacity = 0.5; // Only iOSShake.setShakeTheme(shakeTheme);
Custom font
Shake can be configured to use custom font from your application,
you just have to set font path as a ShakeTheme
font property like shown in the example above.
Before you can use custom font for Shake, you should add custom font to your project.
Dark and light mode
If you want to create a different appearance for the dark and light mode, you should call Shake.setShakeTheme
with desired configuration when app mode is changed.
Here's an example of component you can use to observe dark and light mode changes. Feel free to adjust this component and use it as a root in your project.
import 'package:flutter/cupertino.dart';import 'package:shake_flutter/models/shake_theme.dart';import 'package:shake_flutter/shake_flutter.dart';class DarkModeObserver extends StatefulWidget {final Widget child;DarkModeObserver({required this.child});_DarkModeObserverState createState() => _DarkModeObserverState();}class _DarkModeObserverState extends State<DarkModeObserver>with WidgetsBindingObserver {void initState() {super.initState();WidgetsBinding.instance.addObserver(this);WidgetsBinding.instance.addPostFrameCallback((_) => setShakeTheme());}void didChangePlatformBrightness() {super.didChangePlatformBrightness();setShakeTheme();}void setShakeTheme() {Brightness currentBrightness =View.of(context).platformDispatcher.platformBrightness;if (currentBrightness == Brightness.dark) {ShakeTheme darkTheme = ShakeTheme();darkTheme.accentColor = "#FFFFFF";Shake.setShakeTheme(darkTheme);} else {ShakeTheme lightTheme = ShakeTheme();lightTheme.accentColor = "#000000";Shake.setShakeTheme(lightTheme);}}void dispose() {WidgetsBinding.instance.removeObserver(this);super.dispose();}Widget build(BuildContext context) {return widget.child;}}
Changing the default theme
Sometimes you'll want to change just a specific color from the default theme. Let's say you want to change the default accent color on the screens but wants to keep all other default colors.
You can do it like shown in the example below:
ShakeTheme shakeTheme = ShakeTheme();shakeTheme.accentColor = '#ff0000';Shake.setShakeTheme(shakeTheme);